Design Patterns in Unity3D #5 - Strategy
- Todo Daniel Sidabalok
- Dec 2, 2017
- 3 min read
In a game, we notice that every game objects, even when they are in the same category, have different functionalities and behaviours. The question that is, what happened if we have thousands of objects in, let's say, a single scene? Assuming that all of them share the same base class but they are instances of different subclasses, do we have to override their method one-by-one, given the fact that they have one abstract method?
To solve this problem, let's introduce ourselves with the Strategy pattern. So what does this pattern do is it encapsulates a certain behaviour into an object and it lets every client to choose which behaviour object that it want to use.
In this example, we're gonna look how Strategy pattern works in a game player's skill system, which will show that every skill are be able to choose what kind of skill effect does it want to have both passively and when its activated. With that being said, let's go into it.
1. Create a C# script called SkillBehaviour. Change it from being a class to an interface.
2. Create a C# script called SkillUser and mark it as abstract, because we only want its subclass instances to be exist in the scene later on.
3. Add TriggerActiveEffect() and TriggerPassiveEffect() function into SkillBehaviour with SkillUser parameter

4. In the SkillUser, we are going to store list of skills and its corresponding key inputs. For this tutorial, the skill user will only have one attribute, which is the speed.
5. We will give SkillUser the ability to modify its speed and move forward. These two methods will be used in the concrete SkillBehaviour.
6. Add another 2 new methods AddSkillAndKey to register a skill with the corresponding key input, and ListenToPlayerInput, which is basically checks if one of the key codes in the list is being pressed.
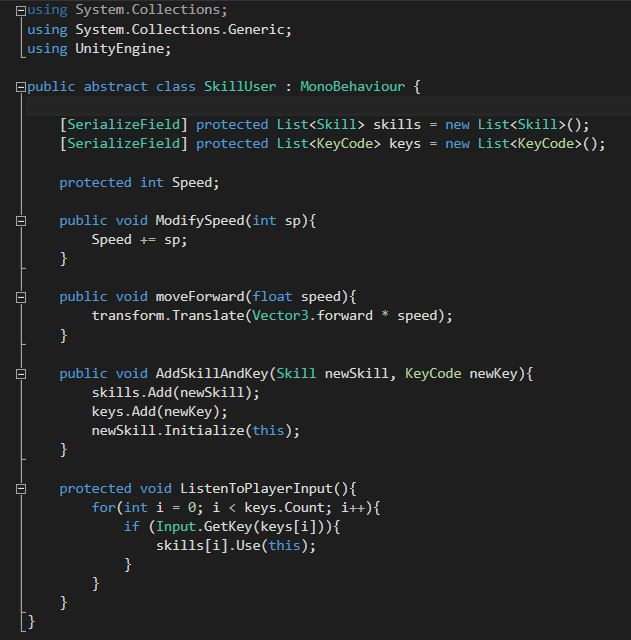
7. Now let's create the Skill class. As I've said before, the skill has a SkillBehaviour inside it.
8. Create two methods, Initialize and Use, both having SkillUser parameter. Initialize is used to trigger the passive effect of the skill behaviour, and Use is used to trigger the active effect of the skill behaviour.
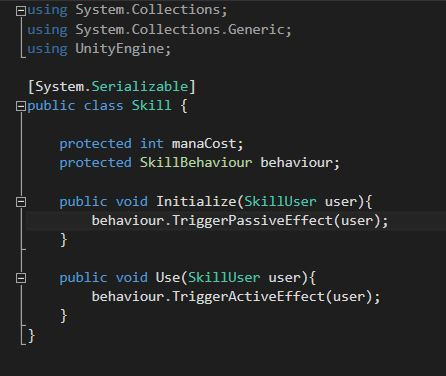
9. Create a script called Summonbehaviour. This is will be our first concrete SkillBehaviour class. As the name states, it summons an object. It doesn't have any passive effect, so just leave the method empty.
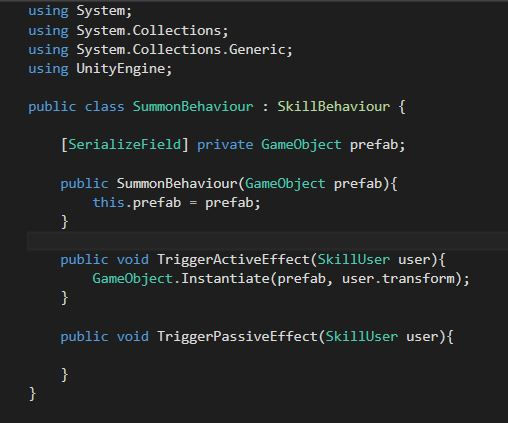
10. Create another concrete class of Skillbehaviour, called BlinkBehaviour. Its active effect blinks the user forward, and its passive adds the user's speed by 10.
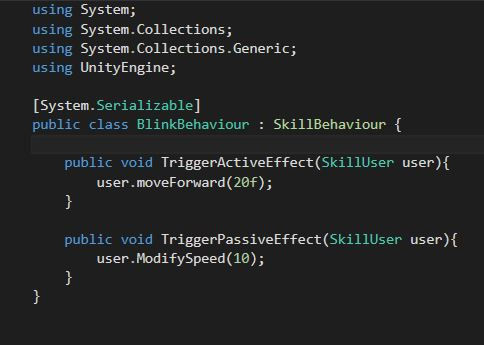
11. Go back to the editor. Create a new sphere and rename it to "Ball Missile". Next, create a folder and name it "Resources". Then, put the ball missile into that folder and delete ball missile from hierarchy.
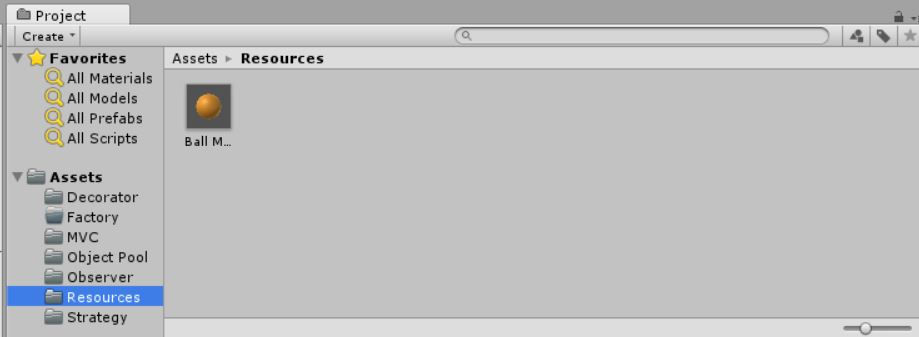
12. Now create a MissileFrenzy script, which is subclass of Skill. In here, we initialize its SkillBehaviour to be the SummonBehaviour, and we specify the ball missile (in the Resources folder) to be the game object that is to be summoned.
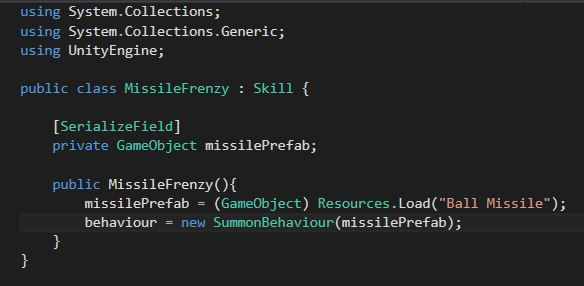
13. Now create another new script called Elusion which is also a subclass of Skill. In this skill, initialize the SkillBehaviour to be the BlinkBehaviour.
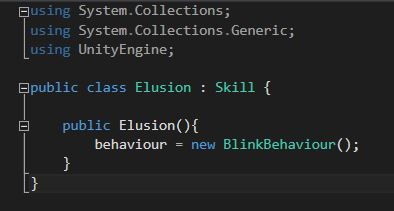
14. Create a new C# script called FPSPlayer, and this will be the concrete SkillUser. In the Start, add the 2 skills that we've made before, and put their corresponding keys to be Alpha 1 and Alpha 2 respectively.
15. In the Update, call the ListenToPlayerInput method to check whether the keys in the list are being pressed.
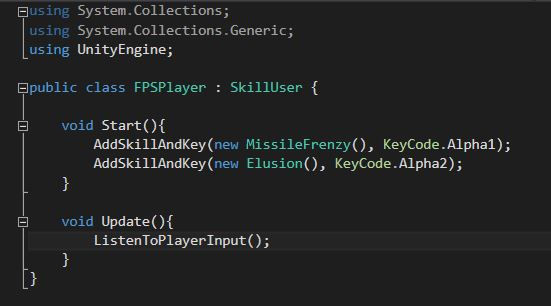
16. Go back to the editor. Attach the FPSPlayer script into the MainCamera.
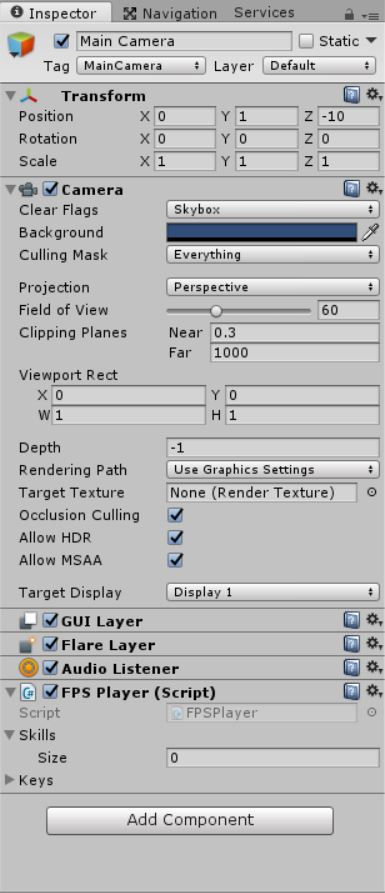
17. Now, click Play. Press button 1 or 2 in your keyboard. What happened? You will spawn a ball missile by pressing 1 and you will blink forward by pressing 2.
So we conclude that we made the Strategy pattern for the player's skill, and that is, we made the skill be able to choose which SkillBehaviour it want to use.
That is all for this tutorial. Hope this helps.
Comments